Building Embedded Wallets Using Tweed
Building Embedded Wallets Using Tweed
Oct 17, 2024
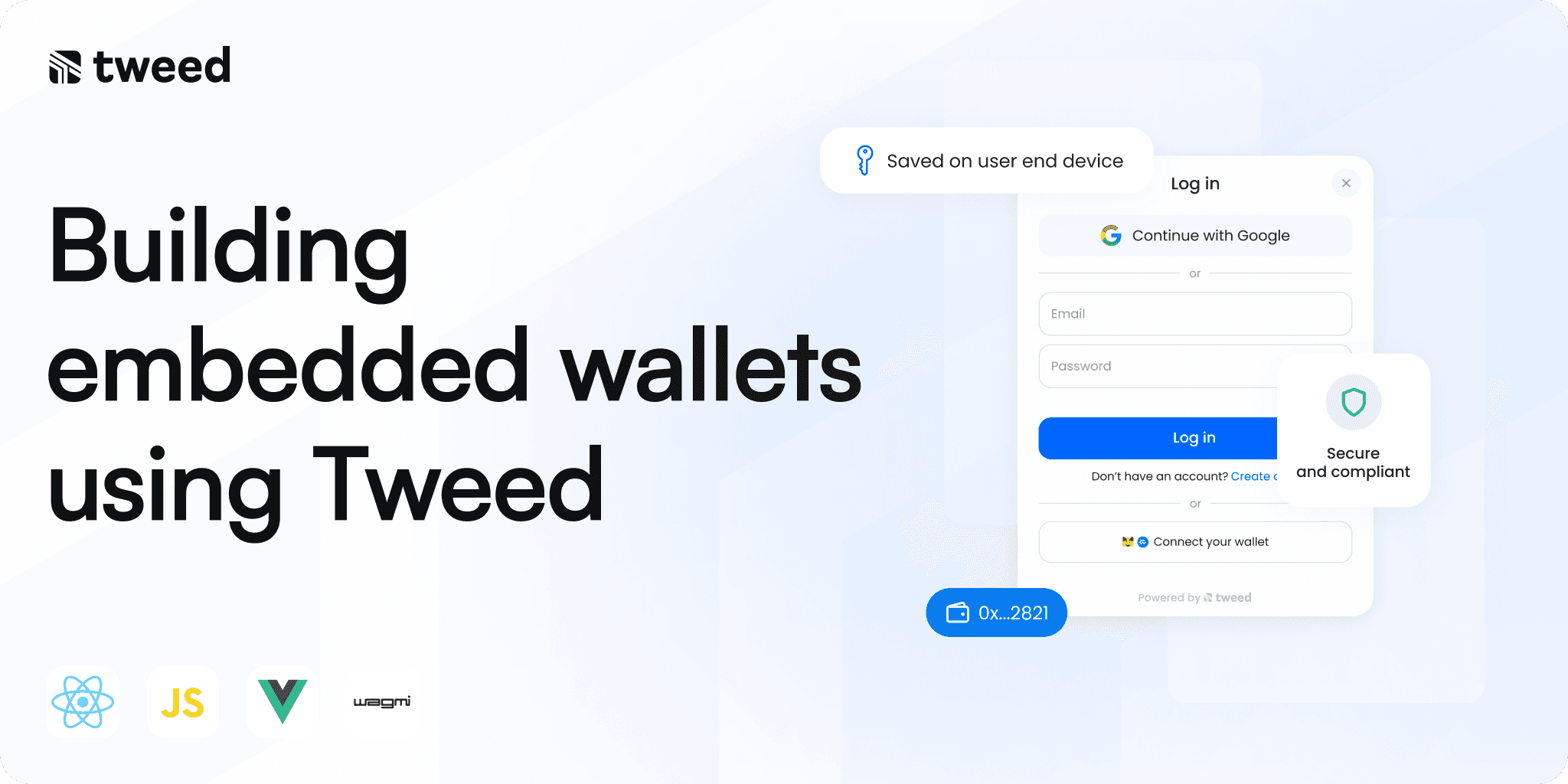
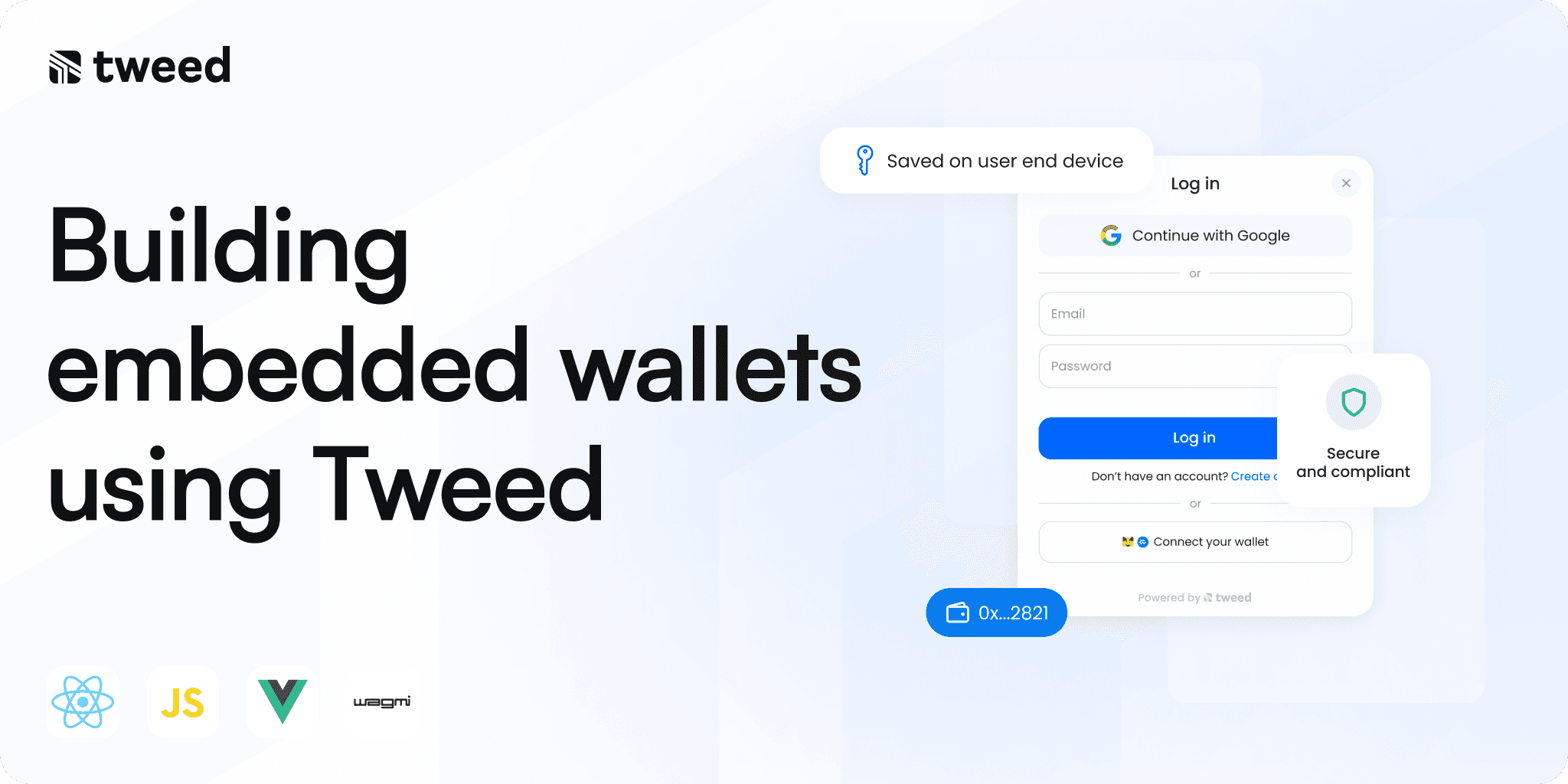
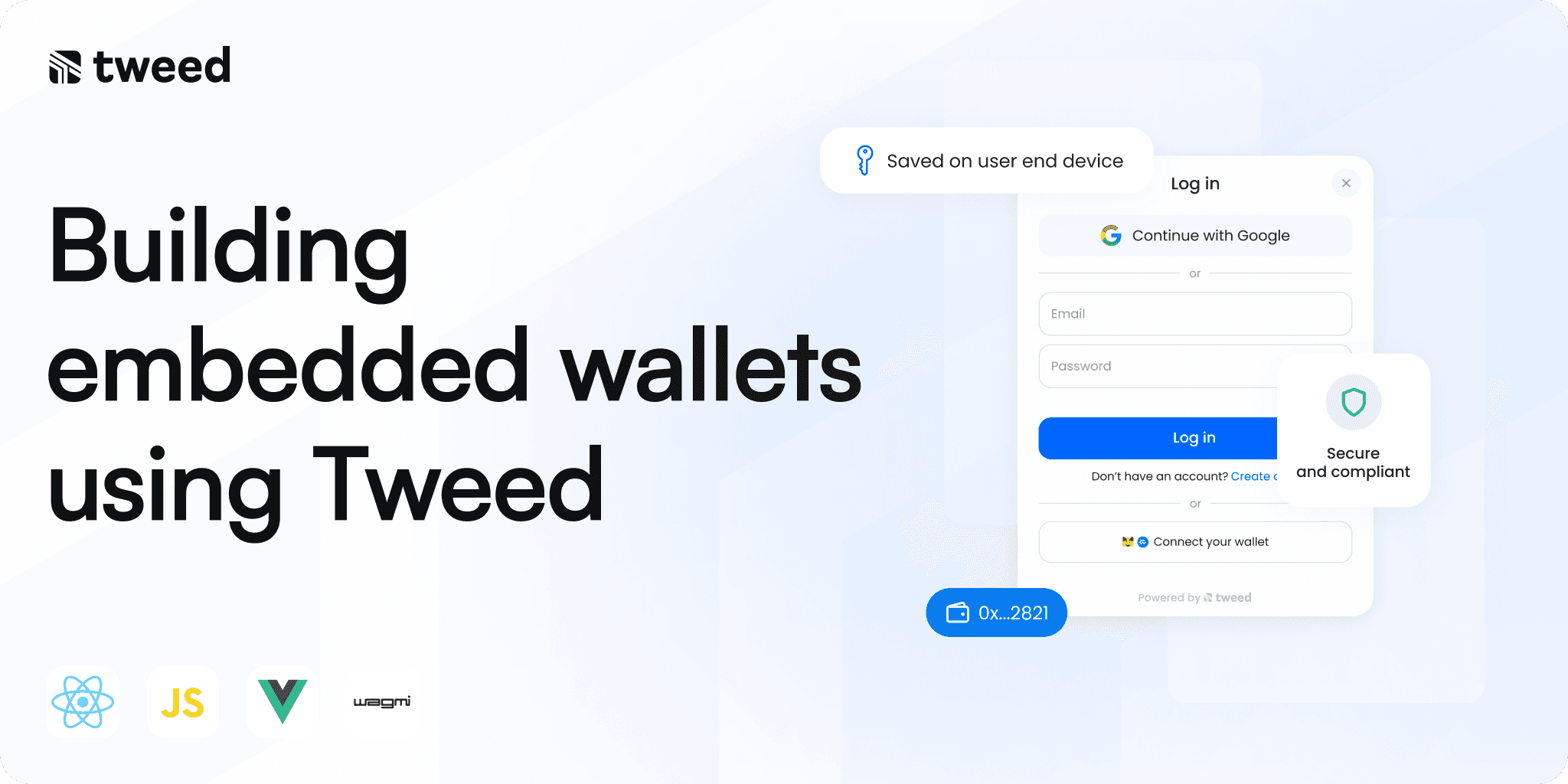
A seamless user experience can make or break an application. Today’s users expect simplicity, whether they’re buying NFTs, making in-app payments, or interacting with DeFi platforms. They don’t want to deal with complicated wallet setups, remembering seed phrases, or switching between apps. This is where embedded wallets shine - offering a fully integrated solution for managing digital assets, directly within an application.
In this article, we'll explore how developers can leverage Tweed's tools to create an embedded wallet that meets the needs of their users.
But First, Let’s Start by Defining an Embedded Wallet
An embedded wallet is a digital wallet that is directly integrated into a website or mobile application. Unlike traditional wallets like MetaMask, which require users to download a plug-in and create an account outside of the main app, embedded wallets allow users to interact in the blockchain directly within the app interface. This means users can buy, sell, send, or receive digital assets without needing to connect to an external wallet.
Tweed’s embedded wallets are non-custodial (where the user retains control of their keys). Using Tweed’s SDK, developers can create their own wallet while offering their users secure and recoverable options for signing transactions, and transferring assets.
Why Use Tweed for Embedded Wallets?
Building an embedded wallet from scratch involves navigating complex technical challenges, from integrating with various blockchains to ensuring compliance and security. Tweed simplifies this process through its robust set of developer tools and APIs.
Here’s why Tweed is the ideal solution for building embedded wallets:
Cross-Chain Compatibility: Tweed supports multiple blockchains, giving developers the flexibility to integrate with various ecosystems all at once.
Simplified Integration: With Tweed’s SDK and API, developers can quickly create wallets, handle transactions, and manage user accounts. This makes it easy to integrate sophisticated wallet functionalities into any app.
Focus on User Experience: Tweed helps developers build intuitive user experiences, focused on mass adoption. Tweed allows for smooth user onboarding through social logins or simplified wallet creation.
Security and Compliance: Tweed incorporates built-in security protocols and compliance features, allowing developers to focus on creating great user experiences while knowing that backend complexities are handled.
Step-by-Step Guide: Building Your Embedded Wallet with Tweed
1. Setting Up the Development Environment
Start by installing Tweed’s SDK and setting up the required dependencies. Here’s a quick start with npm:
npm i @paytweed/core-react ethers
Configure your environment by creating an application in your dashboard account and using your application ID.
2. Add tweed to your React app
install tweed SDK to your React app.
Copy
npm i @paytweed/core-react
3. Integrate Tweed Provider into Your App
Import the Tweed provider from the Tweed React SDK and wrap your application with it. Use the applicationId from the management console.
Add networks
Specify the networks your application will use by passing them to the Tweed provider. Import the Network enum from the React SDK.
import { Network, TweedProvider } from "@paytweed/core-react";
import DemoPage from "pages/demo";
function App() {
return (
<>
<TweedProvider
applicationId="YOUR_APP_ID"
options={{
chains: [Network.POLYGON, Network.ETHEREUM]
}}
>
<HomePage />
</TweedProvider>
</>
);
}
4. Connect the User
Once the Tweed SDK is set up in your application, the first step is to connect the user. Use the connect function from the useAuth hook to display the sign-up/in modal. This allows users to create an account, and a wallet is automatically created for them. You can offer multiple sign-up options; for more details, visit here.
Utilize the loading state from the useTweed hook to manage the loading state, and implement a check to verify that the Tweed client is initialized.
import { useAuth, useTweed } from "@paytweed/core-react";
function HomePage() {
const { connect } = useAuth()
const { client, loading } = useTweed();
function handleConnect() {
if(!client) return
connect()
}
return (
<>
<h1>HomePage</h1>
{ loading ?
<h2> loading... </h2> :
<button onClick={handleConnect}>connect</button>
}
</>
);
}
export default HomePage;
*Token Checkout
If you are integrating WaaS for token checkout, you are done at this point and can return to continue the token checkout integration
5. Create Ethereum Provider
With the user logged in and authenticated, we are able to get the Ethereum provider to handle on-chain actions like sending transactions or checking balances.
import useWeb3 hook from tweed SDK
import { useWeb3, Network } from "@paytweed/core-react";
obtain the Ethereum provider
Create a state to hold the provider and a function to obtain it. In this example, we use the useEffect hook to initialize the provider as soon as the user connects, making it available immediately.
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}d
useEffect(() => {
getProvider();
}, [provider]);
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<button onClick={handleConnect}>connect</button>
)}
</>
);
}
export default HomePage;
6. Test the Integration
Let's create a transaction using the sendTransaction function from ethers.
async function sendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
async function handleSendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<div>
<button onClick={handleConnect}>connect</button>
<button onClick={handleSendTransaction}>send tx.</button>
</div>
)}
</>
);
}
export default HomePage;
Best Practices for Developing with Tweed
Focus on User Experience: Prioritize intuitive onboarding and clear messaging. Use Tweed’s social login options to lower the barrier for new users.
Testing Thoroughly: Use tools like Hardhat for testing Ethereum interactions or Anchor for Solana-based projects. This ensures your wallet behaves correctly in a range of scenarios before going live.
KYC: Make sure you have done proper compliance before going live. You will need to complete KYC/B to go live with Tweed’s Token Checkout solution.
Build Seamless Wallet Experiences with Tweed
Tweed provides a powerful platform for developers looking to integrate embedded wallets into their apps. Its support for multiple blockchains, easy-to-use SDK, and focus on user experience make it an excellent choice for building the next generation of Web3 applications. In addition to EtherJS, you can also use Wagmi libraries with Tweed’s SDK to build your embedded wallet.
With Tweed, you can focus on what matters—creating products that users love—while leaving the complexities of wallet infrastructure behind.
Ready to get started? Dive into our developer console and start building your embedded wallet today!
A seamless user experience can make or break an application. Today’s users expect simplicity, whether they’re buying NFTs, making in-app payments, or interacting with DeFi platforms. They don’t want to deal with complicated wallet setups, remembering seed phrases, or switching between apps. This is where embedded wallets shine - offering a fully integrated solution for managing digital assets, directly within an application.
In this article, we'll explore how developers can leverage Tweed's tools to create an embedded wallet that meets the needs of their users.
But First, Let’s Start by Defining an Embedded Wallet
An embedded wallet is a digital wallet that is directly integrated into a website or mobile application. Unlike traditional wallets like MetaMask, which require users to download a plug-in and create an account outside of the main app, embedded wallets allow users to interact in the blockchain directly within the app interface. This means users can buy, sell, send, or receive digital assets without needing to connect to an external wallet.
Tweed’s embedded wallets are non-custodial (where the user retains control of their keys). Using Tweed’s SDK, developers can create their own wallet while offering their users secure and recoverable options for signing transactions, and transferring assets.
Why Use Tweed for Embedded Wallets?
Building an embedded wallet from scratch involves navigating complex technical challenges, from integrating with various blockchains to ensuring compliance and security. Tweed simplifies this process through its robust set of developer tools and APIs.
Here’s why Tweed is the ideal solution for building embedded wallets:
Cross-Chain Compatibility: Tweed supports multiple blockchains, giving developers the flexibility to integrate with various ecosystems all at once.
Simplified Integration: With Tweed’s SDK and API, developers can quickly create wallets, handle transactions, and manage user accounts. This makes it easy to integrate sophisticated wallet functionalities into any app.
Focus on User Experience: Tweed helps developers build intuitive user experiences, focused on mass adoption. Tweed allows for smooth user onboarding through social logins or simplified wallet creation.
Security and Compliance: Tweed incorporates built-in security protocols and compliance features, allowing developers to focus on creating great user experiences while knowing that backend complexities are handled.
Step-by-Step Guide: Building Your Embedded Wallet with Tweed
1. Setting Up the Development Environment
Start by installing Tweed’s SDK and setting up the required dependencies. Here’s a quick start with npm:
npm i @paytweed/core-react ethers
Configure your environment by creating an application in your dashboard account and using your application ID.
2. Add tweed to your React app
install tweed SDK to your React app.
Copy
npm i @paytweed/core-react
3. Integrate Tweed Provider into Your App
Import the Tweed provider from the Tweed React SDK and wrap your application with it. Use the applicationId from the management console.
Add networks
Specify the networks your application will use by passing them to the Tweed provider. Import the Network enum from the React SDK.
import { Network, TweedProvider } from "@paytweed/core-react";
import DemoPage from "pages/demo";
function App() {
return (
<>
<TweedProvider
applicationId="YOUR_APP_ID"
options={{
chains: [Network.POLYGON, Network.ETHEREUM]
}}
>
<HomePage />
</TweedProvider>
</>
);
}
4. Connect the User
Once the Tweed SDK is set up in your application, the first step is to connect the user. Use the connect function from the useAuth hook to display the sign-up/in modal. This allows users to create an account, and a wallet is automatically created for them. You can offer multiple sign-up options; for more details, visit here.
Utilize the loading state from the useTweed hook to manage the loading state, and implement a check to verify that the Tweed client is initialized.
import { useAuth, useTweed } from "@paytweed/core-react";
function HomePage() {
const { connect } = useAuth()
const { client, loading } = useTweed();
function handleConnect() {
if(!client) return
connect()
}
return (
<>
<h1>HomePage</h1>
{ loading ?
<h2> loading... </h2> :
<button onClick={handleConnect}>connect</button>
}
</>
);
}
export default HomePage;
*Token Checkout
If you are integrating WaaS for token checkout, you are done at this point and can return to continue the token checkout integration
5. Create Ethereum Provider
With the user logged in and authenticated, we are able to get the Ethereum provider to handle on-chain actions like sending transactions or checking balances.
import useWeb3 hook from tweed SDK
import { useWeb3, Network } from "@paytweed/core-react";
obtain the Ethereum provider
Create a state to hold the provider and a function to obtain it. In this example, we use the useEffect hook to initialize the provider as soon as the user connects, making it available immediately.
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}d
useEffect(() => {
getProvider();
}, [provider]);
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<button onClick={handleConnect}>connect</button>
)}
</>
);
}
export default HomePage;
6. Test the Integration
Let's create a transaction using the sendTransaction function from ethers.
async function sendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
async function handleSendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<div>
<button onClick={handleConnect}>connect</button>
<button onClick={handleSendTransaction}>send tx.</button>
</div>
)}
</>
);
}
export default HomePage;
Best Practices for Developing with Tweed
Focus on User Experience: Prioritize intuitive onboarding and clear messaging. Use Tweed’s social login options to lower the barrier for new users.
Testing Thoroughly: Use tools like Hardhat for testing Ethereum interactions or Anchor for Solana-based projects. This ensures your wallet behaves correctly in a range of scenarios before going live.
KYC: Make sure you have done proper compliance before going live. You will need to complete KYC/B to go live with Tweed’s Token Checkout solution.
Build Seamless Wallet Experiences with Tweed
Tweed provides a powerful platform for developers looking to integrate embedded wallets into their apps. Its support for multiple blockchains, easy-to-use SDK, and focus on user experience make it an excellent choice for building the next generation of Web3 applications. In addition to EtherJS, you can also use Wagmi libraries with Tweed’s SDK to build your embedded wallet.
With Tweed, you can focus on what matters—creating products that users love—while leaving the complexities of wallet infrastructure behind.
Ready to get started? Dive into our developer console and start building your embedded wallet today!
A seamless user experience can make or break an application. Today’s users expect simplicity, whether they’re buying NFTs, making in-app payments, or interacting with DeFi platforms. They don’t want to deal with complicated wallet setups, remembering seed phrases, or switching between apps. This is where embedded wallets shine - offering a fully integrated solution for managing digital assets, directly within an application.
In this article, we'll explore how developers can leverage Tweed's tools to create an embedded wallet that meets the needs of their users.
But First, Let’s Start by Defining an Embedded Wallet
An embedded wallet is a digital wallet that is directly integrated into a website or mobile application. Unlike traditional wallets like MetaMask, which require users to download a plug-in and create an account outside of the main app, embedded wallets allow users to interact in the blockchain directly within the app interface. This means users can buy, sell, send, or receive digital assets without needing to connect to an external wallet.
Tweed’s embedded wallets are non-custodial (where the user retains control of their keys). Using Tweed’s SDK, developers can create their own wallet while offering their users secure and recoverable options for signing transactions, and transferring assets.
Why Use Tweed for Embedded Wallets?
Building an embedded wallet from scratch involves navigating complex technical challenges, from integrating with various blockchains to ensuring compliance and security. Tweed simplifies this process through its robust set of developer tools and APIs.
Here’s why Tweed is the ideal solution for building embedded wallets:
Cross-Chain Compatibility: Tweed supports multiple blockchains, giving developers the flexibility to integrate with various ecosystems all at once.
Simplified Integration: With Tweed’s SDK and API, developers can quickly create wallets, handle transactions, and manage user accounts. This makes it easy to integrate sophisticated wallet functionalities into any app.
Focus on User Experience: Tweed helps developers build intuitive user experiences, focused on mass adoption. Tweed allows for smooth user onboarding through social logins or simplified wallet creation.
Security and Compliance: Tweed incorporates built-in security protocols and compliance features, allowing developers to focus on creating great user experiences while knowing that backend complexities are handled.
Step-by-Step Guide: Building Your Embedded Wallet with Tweed
1. Setting Up the Development Environment
Start by installing Tweed’s SDK and setting up the required dependencies. Here’s a quick start with npm:
npm i @paytweed/core-react ethers
Configure your environment by creating an application in your dashboard account and using your application ID.
2. Add tweed to your React app
install tweed SDK to your React app.
Copy
npm i @paytweed/core-react
3. Integrate Tweed Provider into Your App
Import the Tweed provider from the Tweed React SDK and wrap your application with it. Use the applicationId from the management console.
Add networks
Specify the networks your application will use by passing them to the Tweed provider. Import the Network enum from the React SDK.
import { Network, TweedProvider } from "@paytweed/core-react";
import DemoPage from "pages/demo";
function App() {
return (
<>
<TweedProvider
applicationId="YOUR_APP_ID"
options={{
chains: [Network.POLYGON, Network.ETHEREUM]
}}
>
<HomePage />
</TweedProvider>
</>
);
}
4. Connect the User
Once the Tweed SDK is set up in your application, the first step is to connect the user. Use the connect function from the useAuth hook to display the sign-up/in modal. This allows users to create an account, and a wallet is automatically created for them. You can offer multiple sign-up options; for more details, visit here.
Utilize the loading state from the useTweed hook to manage the loading state, and implement a check to verify that the Tweed client is initialized.
import { useAuth, useTweed } from "@paytweed/core-react";
function HomePage() {
const { connect } = useAuth()
const { client, loading } = useTweed();
function handleConnect() {
if(!client) return
connect()
}
return (
<>
<h1>HomePage</h1>
{ loading ?
<h2> loading... </h2> :
<button onClick={handleConnect}>connect</button>
}
</>
);
}
export default HomePage;
*Token Checkout
If you are integrating WaaS for token checkout, you are done at this point and can return to continue the token checkout integration
5. Create Ethereum Provider
With the user logged in and authenticated, we are able to get the Ethereum provider to handle on-chain actions like sending transactions or checking balances.
import useWeb3 hook from tweed SDK
import { useWeb3, Network } from "@paytweed/core-react";
obtain the Ethereum provider
Create a state to hold the provider and a function to obtain it. In this example, we use the useEffect hook to initialize the provider as soon as the user connects, making it available immediately.
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}d
useEffect(() => {
getProvider();
}, [provider]);
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<button onClick={handleConnect}>connect</button>
)}
</>
);
}
export default HomePage;
6. Test the Integration
Let's create a transaction using the sendTransaction function from ethers.
async function sendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
Updating Our Page with the New Code
import { useAuth, useTweed, useWeb3, Network } from "@paytweed/core-react";
import { BrowserProvider } from "ethers";
import { useState, useEffect } from "react";
function HomePage() {
const { connect } = useAuth();
const { client, loading } = useTweed();
const { getEthereumProvider } = useWeb3();
const [provider, setProvider] = useState<BrowserProvider | null>(null);
async function getProvider() {
const provider = await getEthereumProvider(Network.ETHEREUM_SEPOLIA);
const web3provider = new BrowserProvider(provider);
setProvider(web3provider);
}
useEffect(() => {
getProvider();
}, [provider]);
function handleConnect() {
if (!client) return;
connect();
}
async function handleSendTransaction() {
if (!provider) return;
const signer = await provider.getSigner();
const txHash = await signer.sendTransaction({
to: "0x03928fc2FD91491919F55C8658477fE1352338E7",
value: "0.1",
});
console.log('transaction sent', txHash);
}
return (
<>
<h1>HomePage</h1>
{loading ? (
<h2>loading...</h2>
) : (
<div>
<button onClick={handleConnect}>connect</button>
<button onClick={handleSendTransaction}>send tx.</button>
</div>
)}
</>
);
}
export default HomePage;
Best Practices for Developing with Tweed
Focus on User Experience: Prioritize intuitive onboarding and clear messaging. Use Tweed’s social login options to lower the barrier for new users.
Testing Thoroughly: Use tools like Hardhat for testing Ethereum interactions or Anchor for Solana-based projects. This ensures your wallet behaves correctly in a range of scenarios before going live.
KYC: Make sure you have done proper compliance before going live. You will need to complete KYC/B to go live with Tweed’s Token Checkout solution.
Build Seamless Wallet Experiences with Tweed
Tweed provides a powerful platform for developers looking to integrate embedded wallets into their apps. Its support for multiple blockchains, easy-to-use SDK, and focus on user experience make it an excellent choice for building the next generation of Web3 applications. In addition to EtherJS, you can also use Wagmi libraries with Tweed’s SDK to build your embedded wallet.
With Tweed, you can focus on what matters—creating products that users love—while leaving the complexities of wallet infrastructure behind.
Ready to get started? Dive into our developer console and start building your embedded wallet today!